-
Notifications
You must be signed in to change notification settings - Fork 3
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Jobs/Burst based substepping #25
Comments
Inclusion of radiation evaluationLike irradiances, radiation evaluation is also subject to huge inaccuracies at high timewarp rates. Ideally, we should move it to the substep simulation. Kerbalism4/src/Kerbalism/Database/VesselData/VesselData.cs Lines 979 to 1002 in 1e390eb
In terms of final output, this translate into getting substepped averages (instead of "current position") for the following VesselData radiation rates (rad/s):
The current code is a bit messy. The core method to port is the Kerbalism4/src/Kerbalism/Radiation/Radiation.cs Lines 618 to 770 in 1e390eb
You will notice that for each of the above variable, there is a matching boolean. This is because we used to only output the "total" aggregate radiation, and booleans to identify what sources were "active". I quick-and-dirty changed that recently so we have access to the detail rate of each source, but I was also in the process of refactoring the whole thing so In terms of re-implementation, a quick overview : You will need to make a "job-safe" copy of the Belts and magnetopause radiation are computed by evaluating the vessel position against the fields shape, which are represented by math functions. The called methods are a bit all over the place for no reason other than technical debt, but you should be able to rebuild the execution path quite easily by following the execution of Solar/bodies radiation are directional, and affected by other bodies occlusion (and also, they are the same value in RadiationModel). From a physics POV, they are identical to the solar irradiance, so you can likely just merge that into your existing code. Storm radiation is a bit trickier. As mentioned in #5, I intended to more or less entirely scrap the current per-vessel-random implementation, possibly in favor of generating "cone" storms, where every vessel/body inside the cone is subject to the storm. For now, I would suggest to postpone including storm radiation in the substep sim until this is done. |
beloved modders, i just found out about kerbalism 4. are you still working on it? Cheers! |
Replace the managed thread based substepping sim with a Jobs/Burst implementation.
Overview of the substep sim :
In
SubStepSim.OnFixedUpdate()
(called byKerbalism.FixedUpdate()
before anything else has been done) :maxFutureUT
, the maximum time in the future that might be attained in the next fixedupdate at max timewarp :currentUT + (TimeWarp.fetch.warpRates[7] * 0.02) + one extra substep just in case
maxFutureUT
Later in
Kerbalism.FixedUpdate()
,VesselData.EnvironmentUpdate
will be called.The
elapsedSeconds
parameter is checked against the substep duration "constant" (60s for example).If
elapsedSecond < substepDuration * 2.0
:SubStepSim.OnFixedUpdate()
SubStepSim.OnFixedUpdate()
)else
SubStepSim.OnFixedUpdate()
Data : current implementation performance
Save : 20 vessels.zip
Profiler results (2C/[email protected], Cinebench R20 MT : 1135 / ST : 453) :
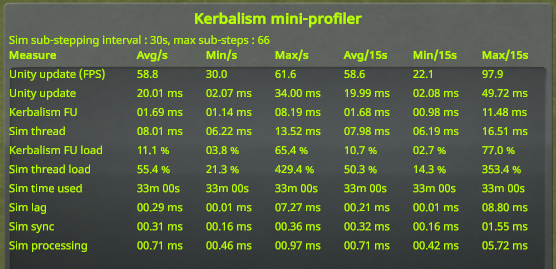
Relevant metrics :
The text was updated successfully, but these errors were encountered: