|
1 |
| -# example |
| 1 | +# App Upgrade: Flutter SDK |
2 | 2 |
|
3 |
| -A new Flutter project. |
| 3 | +Flutter SDK for [App Upgrade](https://appupgrade.dev) |
4 | 4 |
|
5 |
| -## Getting Started |
| 5 | +App Upgrade is a service let your users know when to upgrade your apps or force them to upgrade the app. |
6 | 6 |
|
7 |
| -This project is a starting point for a Flutter application. |
| 7 | +[](https://twitter.com/app_upgrade) |
| 8 | +[](https://www.youtube.com/channel/UC0ZVJPYHFVuMwEsro4VZKXw) |
8 | 9 |
|
9 |
| -A few resources to get you started if this is your first Flutter project: |
| 10 | +Many times we need to force upgrade mobile apps on users' mobile. Having faced this issue multiple times decided to find a better way to tackle this problem. After doing some research on how people are doing this there are so many custom solutions or checking with the play store or AppStore API if there is a new version available. Although this works if we just want to nudge users that there is a new version available. It doesn't solve the problem where we want to make a decision.. whether it's a soft graceful update or we want to force update. So here is this product that will make developers' life easy. We can set custom messages.. see the versions in beautify dashboard, and many exciting features in the roadmap ahead. |
10 | 11 |
|
11 |
| -- [Lab: Write your first Flutter app](https://docs.flutter.dev/get-started/codelab) |
12 |
| -- [Cookbook: Useful Flutter samples](https://docs.flutter.dev/cookbook) |
| 12 | +## Installation |
| 13 | +With flutter |
| 14 | +``` |
| 15 | +flutter pub add app_upgrade_flutter_sdk |
| 16 | +``` |
13 | 17 |
|
14 |
| -For help getting started with Flutter development, view the |
15 |
| -[online documentation](https://docs.flutter.dev/), which offers tutorials, |
16 |
| -samples, guidance on mobile development, and a full API reference. |
| 18 | +Import the package |
| 19 | +``` |
| 20 | +import 'package:app_upgrade_flutter_sdk/app_upgrade_flutter_sdk.dart'; |
| 21 | +``` |
| 22 | + |
| 23 | +## How to use it. |
| 24 | +1. Register on App Upgrade and follow the instructions to create project and get the x-api-key. |
| 25 | + |
| 26 | +2. Wrap the body widget with the `AppUpgradeAlert` widget, provide x-api-key, appInfo of your app and rest will be handled. |
| 27 | +```dart |
| 28 | +import 'package:flutter/material.dart'; |
| 29 | +import 'package:upgrader/upgrader.dart'; |
| 30 | +
|
| 31 | +void main() => runApp(MyApp()); |
| 32 | +
|
| 33 | +class MyApp extends StatelessWidget { |
| 34 | + MyApp({ |
| 35 | + Key key, |
| 36 | + }) : super(key: key); |
| 37 | +
|
| 38 | + @override |
| 39 | + Widget build(BuildContext context) { |
| 40 | + |
| 41 | + AppInfo appInfo = AppInfo( |
| 42 | + appName: 'Wallpaper app', // Your app name |
| 43 | + appVersion: '1.0.0', // Your app version |
| 44 | + platform: 'android', // App Platform, android or ios |
| 45 | + environment: 'production', // Environment in which app is running, production, staging or development etc. |
| 46 | + appLanguage: 'en' // App language ex: en, es etc. Optional. |
| 47 | + ); |
| 48 | + |
| 49 | + return MaterialApp( |
| 50 | + title: 'App Upgrade Flutter Example', |
| 51 | + home: Scaffold( |
| 52 | + appBar: AppBar( |
| 53 | + title: Text('App Upgrade Flutter Example'), |
| 54 | + ), |
| 55 | + body: AppUpgradeAlert( |
| 56 | + xApiKey: 'ZWY0ZDhjYjgtYThmMC00NTg5LWI0NmUtMjM5OWZkNjkzMzQ5', // Your x-api-key |
| 57 | + appInfo: appInfo, |
| 58 | + child: Center(child: Text('Hello World!')), |
| 59 | + ) |
| 60 | + ), |
| 61 | + ); |
| 62 | + } |
| 63 | +} |
| 64 | +``` |
| 65 | + |
| 66 | +Optionally you can also provide dialog cofiguration such as dialogStyle (material or cupertino). Full details is below. |
| 67 | + |
| 68 | +``` |
| 69 | + dialogStyle: DialogStyle.material, // cupertino or material, default is material |
| 70 | + title: 'App update required!', // Title that will be shown in the Diaglog. Default is "App Update Required!" |
| 71 | + updateButtonTitle: 'Update Now', // Update button title. Default is "Update" |
| 72 | + laterButtonTitle: 'Later' // Later button title. Default is "Later" |
| 73 | +``` |
| 74 | + |
| 75 | +Example with Dialog Config: |
| 76 | +```dart |
| 77 | +import 'package:flutter/material.dart'; |
| 78 | +import 'package:upgrader/upgrader.dart'; |
| 79 | +
|
| 80 | +void main() => runApp(MyApp()); |
| 81 | +
|
| 82 | +class MyApp extends StatelessWidget { |
| 83 | + MyApp({ |
| 84 | + Key key, |
| 85 | + }) : super(key: key); |
| 86 | +
|
| 87 | + @override |
| 88 | + Widget build(BuildContext context) { |
| 89 | + |
| 90 | + AppInfo appInfo = AppInfo( |
| 91 | + appName: 'Wallpaper app', |
| 92 | + appVersion: '1.0.0', |
| 93 | + platform: 'android', |
| 94 | + environment: 'production', |
| 95 | + appLanguage: 'en' // App language ex: en, es etc. Optional. |
| 96 | + ); |
| 97 | + |
| 98 | + DialogConfig dialogConfig = DialogConfig( |
| 99 | + dialogStyle: DialogStyle.material, |
| 100 | + title: 'App update required!', |
| 101 | + updateButtonTitle: 'Update Now', |
| 102 | + laterButtonTitle: 'Later' |
| 103 | + ); |
| 104 | + |
| 105 | + return MaterialApp( |
| 106 | + title: 'App Upgrade Flutter Example', |
| 107 | + home: Scaffold( |
| 108 | + appBar: AppBar( |
| 109 | + title: Text('App Upgrade Flutter Example'), |
| 110 | + ), |
| 111 | + body: AppUpgradeAlert( |
| 112 | + xApiKey: 'MmQwMDU3YWEtNmEzOC00NjQ4LThlYWItNWQ4YTI3YzZdfjdkfdkfdg5', |
| 113 | + appInfo: appInfo, |
| 114 | + dialogConfig: dialogConfig, |
| 115 | + child: Center(child: Text('Hello World!')), |
| 116 | + ) |
| 117 | + ), |
| 118 | + ); |
| 119 | + } |
| 120 | +} |
| 121 | +``` |
| 122 | + |
| 123 | +### Note: |
| 124 | +1. For opening the app store/playstore the app should be live. |
| 125 | +2. It might not be able to open the app store/playstore in simulator. You can try it in physical device. |
| 126 | +3. You can find a sample app from here [app_upgrade_flutter_demo_app](https://github.com/appupgrade-dev/app_upgrade_flutter_demo_app) |
| 127 | +4. Read detailed blog on how to integrate from here [How to upgrade/force upgrade Flutter app](https://appupgrade.dev/blog/how-to-force-upgrade-flutter-app) |
| 128 | + |
| 129 | +## Screenshot of alert - material |
| 130 | + |
| 131 | +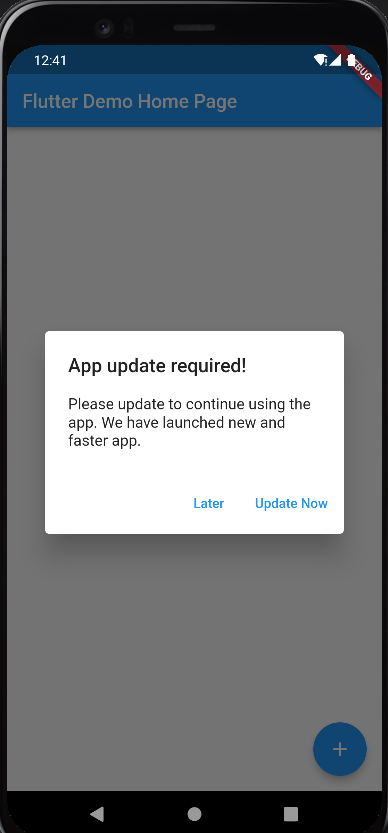 |
| 132 | + |
| 133 | +## Screenshot of Cupertino alert |
| 134 | + |
| 135 | +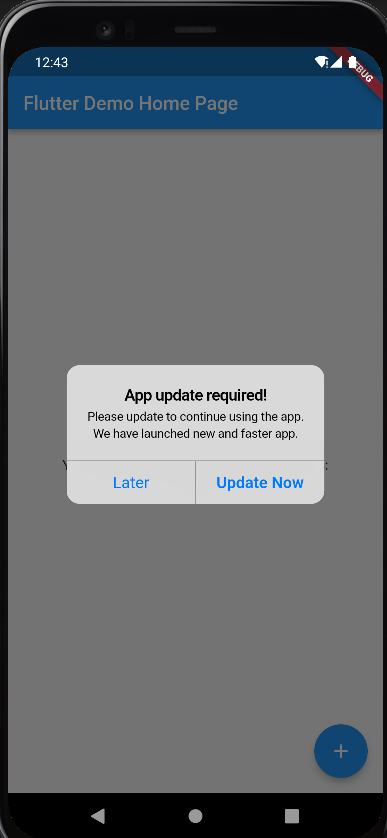 |
| 136 | + |
| 137 | +## Force upgrade screenshot Example |
| 138 | +Only update button is enable. User cannot skip it. |
| 139 | + |
| 140 | +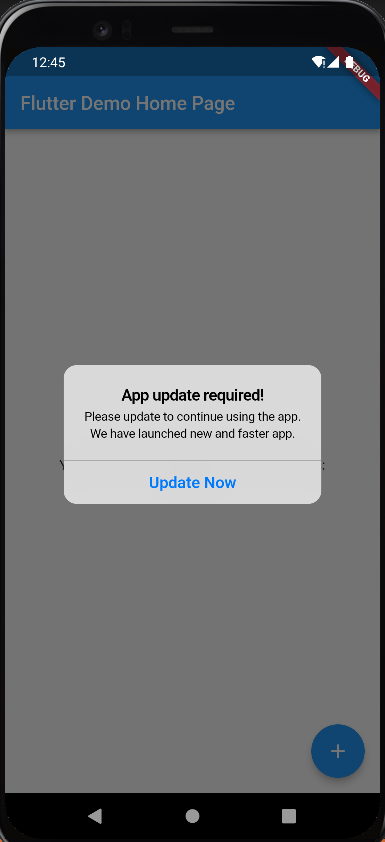 |
| 141 | + |
| 142 | +### Debugging |
| 143 | +You can provide debug true to enable debug logs |
| 144 | +```dart |
| 145 | +import 'package:flutter/material.dart'; |
| 146 | +import 'package:upgrader/upgrader.dart'; |
| 147 | +
|
| 148 | +void main() => runApp(MyApp()); |
| 149 | +
|
| 150 | +class MyApp extends StatelessWidget { |
| 151 | + MyApp({ |
| 152 | + Key key, |
| 153 | + }) : super(key: key); |
| 154 | +
|
| 155 | + @override |
| 156 | + Widget build(BuildContext context) { |
| 157 | + |
| 158 | + AppInfo appInfo = AppInfo( |
| 159 | + appName: 'Wallpaper app', // Your app name |
| 160 | + appVersion: '1.0.0', // Your app version |
| 161 | + platform: 'android', // App Platform, android or ios |
| 162 | + environment: 'production', // Environment in which app is running, production, staging or development etc. |
| 163 | + appLanguage: 'en' // App language ex: en, es etc. Optional. |
| 164 | + ); |
| 165 | + |
| 166 | + return MaterialApp( |
| 167 | + title: 'App Upgrade Flutter Example', |
| 168 | + home: Scaffold( |
| 169 | + appBar: AppBar( |
| 170 | + title: Text('App Upgrade Flutter Example'), |
| 171 | + ), |
| 172 | + body: AppUpgradeAlert( |
| 173 | + xApiKey: 'MmQwMDU3YWEtNmEzOC00NjQ4LThlYWItNWQ4YTI3YzZdfjdkfdkfdg5', // Your x-api-key |
| 174 | + appInfo: appInfo, |
| 175 | + debug: true, // You can specify debug true to print debug logs |
| 176 | + child: Center(child: Text('Hello World!')), |
| 177 | + ) |
| 178 | + ), |
| 179 | + ); |
| 180 | + } |
| 181 | +} |
| 182 | +``` |
| 183 | + |
| 184 | +## App Upgrade Docs |
| 185 | +For more information visit [App Upgrade](https://appupgrade.dev) |
| 186 | + |
| 187 | +### Changelog |
| 188 | + |
| 189 | +Please see [CHANGELOG](CHANGELOG.md) for more information what has changed recently. |
| 190 | + |
| 191 | +### Contributing |
| 192 | + |
| 193 | +Please see [CONTRIBUTING](CONTRIBUTING.md) and [CODE OF CONDUCT](CODE_OF_CONDUCT.md) for details. |
| 194 | + |
| 195 | +### License |
| 196 | + |
| 197 | +The MIT License (MIT). Please see [License File](LICENSE) for more information. |
| 198 | + |
| 199 | +## Need help? |
| 200 | + |
| 201 | +If you're looking for help, try our [Documentation](https://appupgrade.dev/docs/) or our [FAQ](https://appupgrade.dev/docs/app-upgrade-faq). |
| 202 | +If you need support please write to us at [email protected] |
| 203 | + |
| 204 | +### Happy Coding!!! |
0 commit comments