|
1 |
| -# Express Middleware |
| 1 | +# Express Middleware :electric_plug: |
| 2 | + |
| 3 | +--- |
| 4 | + |
| 5 | +## What are express middlewares? :nut_and_bolt: |
| 6 | + |
| 7 | +--- |
| 8 | + |
| 9 | +An Express application is essentially a series of middleware function calls. |
| 10 | + |
| 11 | +Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. The next middleware function is commonly denoted by a variable named next. |
| 12 | + |
| 13 | +--- |
| 14 | + |
| 15 | + |
| 16 | + |
| 17 | +--- |
| 18 | + |
| 19 | +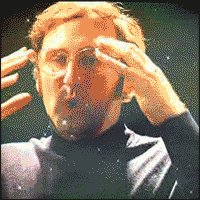 |
| 20 | + |
| 21 | +--- |
| 22 | + |
| 23 | +If the current middleware function does not end the request-response cycle, it must call next() to pass control to the next middleware function. Otherwise, the request will be left hanging. |
| 24 | + |
| 25 | +--- |
| 26 | + |
| 27 | + |
| 28 | + |
| 29 | +--- |
| 30 | + |
| 31 | +## What functionality do they provide?:wrench: |
| 32 | + |
| 33 | +--- |
| 34 | + |
| 35 | +Middleware functions can perform the following tasks: |
| 36 | + |
| 37 | +* Execute any code. |
| 38 | +* Make changes to the request and the response objects. |
| 39 | +* End the request-response cycle. |
| 40 | +* Call the next middleware function in the stack. |
| 41 | + |
| 42 | +--- |
| 43 | + |
| 44 | +An Express application can use the following types of middleware: :clipboard: |
| 45 | + |
| 46 | +1. Application level middleware |
| 47 | +```app.use & app.METHOD``` |
| 48 | +3. Router level middleware |
| 49 | +```router.use & router.METHOD``` |
| 50 | +5. Built-in middleware |
| 51 | +```express.static,express.json,express.urlencoded``` |
| 52 | +7. Error handling middleware |
| 53 | +```app.use(err,req,res,next)``` |
| 54 | +9. Thirdparty middleware |
| 55 | +```bodyparser,cookieparser``` |
| 56 | + |
| 57 | +--- |
| 58 | + |
| 59 | + |
| 60 | +:construction_worker: These middleware and libraries are officially supported by the Connect/Express team: |
| 61 | + |
| 62 | +--- |
| 63 | + |
| 64 | + |
| 65 | + |
| 66 | + |
| 67 | + |
| 68 | +--- |
| 69 | + |
| 70 | + |
| 71 | + |
| 72 | + |
| 73 | +--- |
| 74 | + |
| 75 | +These are some additional popular (third party) middleware modules: |
| 76 | + |
| 77 | + |
| 78 | + |
| 79 | + |
| 80 | + |
| 81 | + |
| 82 | + |
| 83 | +--- |
| 84 | + |
| 85 | + |
| 86 | +## What are some examples of useful express middleware and how do you use them |
| 87 | + |
| 88 | +--- |
| 89 | + |
| 90 | +## Third-party middleware :cop: |
| 91 | + |
| 92 | +--- |
| 93 | + |
| 94 | +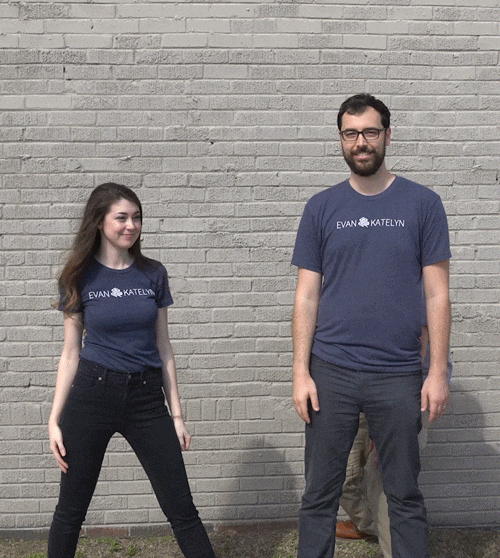 |
| 95 | + |
| 96 | +--- |
| 97 | + |
| 98 | +```javascript |
| 99 | +let cp = require('child_process'); |
| 100 | +// Forking a separate Node.js processes |
| 101 | +let responseTime = require('response-time'); |
| 102 | +// For code timing checks for performance logging |
| 103 | +let assert = require('assert'); |
| 104 | +// assert testing of values |
| 105 | +let helmet = require('helmet'); |
| 106 | +// Helmet module for HTTP header hack mitigations |
| 107 | +let RateLimit = require('express-rate-limit'); |
| 108 | +// IP based rate limiter |
| 109 | +let csp = require('helmet-csp'); |
| 110 | +//Content Security Policy helps prevent unwanted content being injected into your webpages |
| 111 | +let cookieParser = require('cookie-parser') |
| 112 | + |
| 113 | +// load the cookie-parsing middleware |
| 114 | +app.use(cookieParser()) |
| 115 | + |
| 116 | +``` |
| 117 | + |
| 118 | +--- |
| 119 | + |
| 120 | +```javascript |
| 121 | +// Apply limits to all requests |
| 122 | +var limiter = new RateLimit({ |
| 123 | + windowMs: 15 * 60 * 1000, // 15 minutes |
| 124 | + max: 100, // limit each IP to 100 requests per windowMs |
| 125 | + delayMs: 0 // disable delaying - full speed until the max limit is reached |
| 126 | +}); |
| 127 | +app.use(limiter); |
| 128 | +``` |
| 129 | + |
| 130 | +--- |
| 131 | + |
| 132 | +```javascript |
| 133 | + |
| 134 | +app.use(helmet()); // Take the defaults to start with |
| 135 | +app.use(csp({ |
| 136 | + // Specify directives for content sources |
| 137 | + directives: { |
| 138 | + defaultSrc: ["'self'"], |
| 139 | + scriptSrc: ["'self'", "'unsafe-inline'", 'ajax.googleapis.com', 'maxcdn.bootstrapcdn.com'], |
| 140 | + styleSrc: ["'self'", "'unsafe-inline'", 'maxcdn.bootstrapcdn.com'], |
| 141 | + fontSrc: ["'self'", 'maxcdn.bootstrapcdn.com'], |
| 142 | + imgSrc: ['*'] |
| 143 | + // reportUri: '/report-violation', |
| 144 | + } |
| 145 | +})); |
| 146 | + |
| 147 | +``` |
| 148 | + |
| 149 | +--- |
| 150 | + |
| 151 | +```javascript |
| 152 | +// Adds an X-Response-Time header to responses to measure response times |
| 153 | +app.use(responseTime()); |
| 154 | + |
| 155 | +``` |
| 156 | + |
| 157 | +--- |
| 158 | + |
| 159 | +```javascript |
| 160 | +// Sets up the response object in routes to contain a body property with an object of what is parsed from a JSON body request payload |
| 161 | +// There is no need for allowing a huge body, it might be some type of attack, so use the limit option |
| 162 | +app.use(bodyParser.json({ limit: '100kb' })); |
| 163 | +``` |
| 164 | + |
| 165 | +--- |
| 166 | + |
| 167 | +```javascript |
| 168 | +// |
| 169 | +// MongoDB database connection initialization |
| 170 | +// |
| 171 | +var db = {}; |
| 172 | +var MongoClient = require('mongodb').MongoClient; |
| 173 | + |
| 174 | +//Use connect method to connect to the Server |
| 175 | +MongoClient.connect(process.env.MONGODB_CONNECT_URL, { useNewUrlParser: true }, function (err, client) { |
| 176 | + assert.equal(null, err); |
| 177 | + db.client = client; |
| 178 | + db.collection = client.db('myDBname').collection('myDBcollectionName'); |
| 179 | + console.log("Connected to MongoDB server"); |
| 180 | +}); |
| 181 | +``` |
| 182 | + |
| 183 | +--- |
| 184 | + |
| 185 | +### Error Handling |
| 186 | + |
| 187 | +--- |
| 188 | + |
| 189 | + |
| 190 | +Error-handling middleware always takes four arguments. You must provide four arguments to identify it as an error-handling middleware function. Even if you don’t need to use the next object, you must specify it to maintain the sytax. Otherwise, the next object will be interpreted as regular middleware and will fail to handle errors. |
| 191 | + |
| 192 | +--- |
| 193 | + |
| 194 | +example: |
| 195 | +```javascript |
| 196 | +exports.server = (err, req, res, next) => { |
| 197 | + res |
| 198 | + .status(500) |
| 199 | + .sendFile(path.join(__dirname, '..', '..', 'public', '500.html')); |
| 200 | +}; |
| 201 | + |
| 202 | +``` |
| 203 | + |
| 204 | +--- |
| 205 | + |
| 206 | +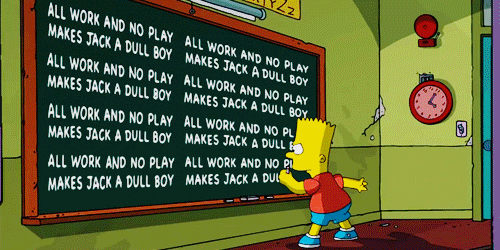 |
| 207 | + |
| 208 | +--- |
| 209 | + |
| 210 | + |
| 211 | +## References: |
| 212 | + |
| 213 | +https://expressjs.com/en/guide/using-middleware.html |
0 commit comments