+
+## Table of Contents
+
+See https://github.com/ipfs-examples/helia-examples#table-of-contents for more information about Helia examples.
+
+## Getting Started
+
+### Prerequisites
+
+https://github.com/ipfs-examples/helia-examples#prerequisites
+
+### Installation and Running example
+
+```console
+> npm install
+> npm run dev
+```
+
+Now open your browser at `http://localhost:5173/`
+
+### Available Scripts
+
+In the project directory, you can run:
+
+#### `npm run dev`
+
+Runs the app in the development mode.
+Open [http://localhost:5173/](http://localhost:5173/) to view it in the browser.
+
+The page will reload if you make edits.
+You will also see any lint errors in the console.
+
+#### `npm run build`
+
+Builds the app for production to the `dist` folder.
+It correctly bundles in production mode and optimizes the build for the best performance.
+
+## Usage
+
+This is a [Remix.js](https://remix.run/) project bootstrapped with [`create-remix@latest`](https://github.com/remix-run/remix/blob/main/packages/create-remix) integrated with `helia`.
+
+You can start editing the page by modifying `app/routes/_index.tsx`. The page auto-updates as you edit the file.
+
+### Learn More
+
+To learn more about Next.js, take a look at the following resources:
+
+- [Remix Documentation](https://remix.run/docs/en/main) - learn about Remix features and API.
+- [Learn Remix](https://remix.run/docs/en/main/start/tutorial) - an interactive Remix tutorial.
+
+You can check out [the Remix GitHub repository](https://github.com/remix-run/remix) - your feedback and contributions are welcome!
+
+### Deploy on Vercel
+
+The easiest way to deploy your Remix app is to use the [Vercel Platform](https://vercel.com/guides/deploying-remix-with-vercel).
+
+Check out our [Remix deployment documentation](https://vercel.com/docs/frameworks/remix) for more details.
+
+_For more examples, please refer to the [Documentation](https://github.com/ipfs-examples/helia-examples#documentation)_
+
+## Documentation
+
+- [IPFS Primer](https://dweb-primer.ipfs.io/)
+- [IPFS Docs](https://docs.ipfs.io/)
+- [Tutorials](https://proto.school)
+- [More examples](https://github.com/ipfs-examples/helia-examples)
+- [API - Helia](https://ipfs.github.io/helia/modules/helia.html)
+- [API - @helia/unixfs](https://ipfs.github.io/helia-unixfs/modules/helia.html)
+
+## Contributing
+
+Contributions are what make the open source community such an amazing place to be learn, inspire, and create. Any contributions you make are **greatly appreciated**.
+
+1. Fork the IPFS Project
+2. Create your Feature Branch (`git checkout -b feature/amazing-feature`)
+3. Commit your Changes (`git commit -a -m 'feat: add some amazing feature'`)
+4. Push to the Branch (`git push origin feature/amazing-feature`)
+5. Open a Pull Request
+
+## Want to hack on IPFS?
+
+[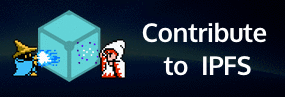](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md)
+
+The IPFS implementation in JavaScript needs your help! There are a few things you can do right now to help out:
+
+Read the [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md) and [JavaScript Contributing Guidelines](https://github.com/ipfs/community/blob/master/CONTRIBUTING_JS.md).
+
+- **Check out existing issues** The [issue list](https://github.com/ipfs/helia/issues) has many that are marked as ['help wanted'](https://github.com/ipfs/helia/issues?q=is%3Aissue+is%3Aopen+sort%3Aupdated-desc+label%3A%22help+wanted%22) or ['difficulty:easy'](https://github.com/ipfs/helia/issues?q=is%3Aissue+is%3Aopen+sort%3Aupdated-desc+label%3Adifficulty%3Aeasy) which make great starting points for development, many of which can be tackled with no prior IPFS knowledge
+- **Look at the [Helia Roadmap](https://github.com/ipfs/helia/blob/main/ROADMAP.md)** This are the high priority items being worked on right now
+- **Perform code reviews** More eyes will help
+ a. speed the project along
+ b. ensure quality, and
+ c. reduce possible future bugs
+- **Add tests**. There can never be enough tests
+
diff --git a/examples/helia-remix/__mocks__/helia.ts b/examples/helia-remix/__mocks__/helia.ts
new file mode 100644
index 00000000..689743d6
--- /dev/null
+++ b/examples/helia-remix/__mocks__/helia.ts
@@ -0,0 +1,6 @@
+export const createHelia = jest.fn().mockResolvedValue({
+ libp2p: {
+ peerId: { toString: () => 'mock-peer-id' },
+ status: 'started'
+ }
+ });
\ No newline at end of file
diff --git a/examples/helia-remix/__tests__/HeliaComponents.test.tsx b/examples/helia-remix/__tests__/HeliaComponents.test.tsx
new file mode 100644
index 00000000..df339123
--- /dev/null
+++ b/examples/helia-remix/__tests__/HeliaComponents.test.tsx
@@ -0,0 +1,24 @@
+import { initHelia } from "../components/helia";
+
+describe('initialize Helia', () => {
+ it('checks if HeliaNode has started', async () => {
+
+ const { heliaNode } = await initHelia();
+
+ expect(heliaNode.libp2p.status).toEqual('started');
+ })
+
+ it('checks if there is a nodeId', async () => {
+
+ const { nodeId } = await initHelia();
+
+ expect(nodeId).toEqual('mock-peer-id');
+ })
+
+ it('checks if nodeId is online', async () => {
+
+ const { nodeIsOnline } = await initHelia();
+
+ expect(nodeIsOnline).toBe(true);
+ })
+})
\ No newline at end of file
diff --git a/examples/helia-remix/app/root.tsx b/examples/helia-remix/app/root.tsx
new file mode 100644
index 00000000..61c8b983
--- /dev/null
+++ b/examples/helia-remix/app/root.tsx
@@ -0,0 +1,45 @@
+import {
+ Links,
+ Meta,
+ Outlet,
+ Scripts,
+ ScrollRestoration,
+} from "@remix-run/react";
+import type { LinksFunction } from "@remix-run/node";
+
+import "./tailwind.css";
+
+export const links: LinksFunction = () => [
+ { rel: "preconnect", href: "https://fonts.googleapis.com" },
+ {
+ rel: "preconnect",
+ href: "https://fonts.gstatic.com",
+ crossOrigin: "anonymous",
+ },
+ {
+ rel: "stylesheet",
+ href: "https://fonts.googleapis.com/css2?family=Inter:ital,opsz,wght@0,14..32,100..900;1,14..32,100..900&display=swap",
+ },
+];
+
+export function Layout({ children }: { children: React.ReactNode }) {
+ return (
+
+
+
+
+
+
+
+
+ {children}
+
+
+
+
+ );
+}
+
+export default function App() {
+ return ;
+}
diff --git a/examples/helia-remix/app/routes/_index.tsx b/examples/helia-remix/app/routes/_index.tsx
new file mode 100644
index 00000000..47f6dae9
--- /dev/null
+++ b/examples/helia-remix/app/routes/_index.tsx
@@ -0,0 +1,169 @@
+import type { MetaFunction } from "@remix-run/node";
+import HeliaComponent from "../../components/helia";
+
+export const meta: MetaFunction = () => {
+ return [
+ { title: "New Helia Remix App" },
+ { name: "description", content: "Welcome to Helia Remix!" },
+ ];
+};
+
+export default function Index() {
+ return (
+