You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
It will be a while before any of this as the current priority is fixing as many bugs as possible.
Some of the ideas, like switching to Pritter, could potentially be done before a breaking change but would need for there not to be any changes being made at the time as it will break any PRs.
Switch ES modules for external API. ES modules will require hiding private modules/methods to prevent non-public APIs from being imported. The easiest way might be to continue to use rollup but have it generate an ES module instead.
There is no backwards compatibility for this, but nearly all supported browsers will support modules once the codebase is updated to ES6. There is only one exception: UC browser, which supports most new features except ES modules.
Putting the code in a module would also prevent older browsers from running it and generating an error which would be a plus.
It would likely need to be split into multiple modules, like:
SCEditor
Default commands (users can then use tree shaking to remove ones they don't want)
BBCode
Default BBCodes (users can then use tree shaking to remove ones they don't want)
XHTML
Plugins in their own modules
ES modules are the future so would be good to use them. It just depends on how important UC browser support is.
Pass in commands and BBCodes via the constructor #
Currently, commands and BBCodes are created using the set() method, which stores them globally, which can be confusing. It also makes it a pain to provide a build of the editor without the default commands.
Making them be passed in means users can load whichever ones they need and pass them in, something like this:
This would make the editor more self-contained and better encapsulate HTML/CSS via the shadow DOM API. All browsers that support ES modules also support Web Components, so support shouldn't be an issue.
Using Web Components could also be used to allow creating the editor declaritavley:
Would need to give commands a title/descriptions and allow mapping shortcuts to command IDs.
Could then make a new command/plugin that can be used to display shortcuts and re-map commands to new shortcuts.
This would give a better UX on non-Latin keyboard layouts as currently triggering a default shortcut requires typing Latin characters.
This would also allow multiple aliases support which could help with typing certain shortcuts (like ctrl+1 that also needs to have ctrl+shift+1 for some French keyboard layouts).
Currently the <code> tag has a lot of special exceptions. They should be made configurable to all tags. It would also be better to change to using <pre><code> and store it as <pre data-sce-code="true"> while editing in WYSIWYG.
Not really possible to provide any backwards compatibility for this.
The utils each method can be replaced with forEach and Array.from, which are now available in all supported browsers.
It might also be worth reducing or outright removing the exposed dom.* functions.
Most of them are either very editor specific or can be done using the native APIs fairly easily.
Alias of skipLastLineBreak which only exists for backwards compatibility. Isn't documented so unlikely anybody is still using it and easy to migrate if anyone is.
Can offer backwards compatibility easily via backwards compatibility plugin.
Can sort of be done currently if there are no attributes but if there are any attributes, it will need to be proper support to work. This can default to current behaviour so can be backwards compatible.
Switch to using Prettier to auto-format the code. Fully backwards compatible and makes it easier to contribute code as contributors don't need to think about formatting.
reacted with thumbs up emoji reacted with thumbs down emoji reacted with laugh emoji reacted with hooray emoji reacted with confused emoji reacted with heart emoji reacted with rocket emoji reacted with eyes emoji
-
Proposal for v4
It will be a while before any of this as the current priority is fixing as many bugs as possible.
Some of the ideas, like switching to Pritter, could potentially be done before a breaking change but would need for there not to be any changes being made at the time as it will break any PRs.
Current ideas:
bbcode.isPreFormatted
Switch to ES modules #
Switch ES modules for external API. ES modules will require hiding private modules/methods to prevent non-public APIs from being imported. The easiest way might be to continue to use rollup but have it generate an ES module instead.
There is no backwards compatibility for this, but nearly all supported browsers will support modules once the codebase is updated to ES6. There is only one exception: UC browser, which supports most new features except ES modules.
Putting the code in a module would also prevent older browsers from running it and generating an error which would be a plus.
It would likely need to be split into multiple modules, like:
ES modules are the future so would be good to use them. It just depends on how important UC browser support is.
Pass in commands and BBCodes via the constructor #
Currently, commands and BBCodes are created using the set() method, which stores them globally, which can be confusing. It also makes it a pain to provide a build of the editor without the default commands.
Making them be passed in means users can load whichever ones they need and pass them in, something like this:
Passing them in via the constructor should make it obvious which ones are loaded and where they originate.
Can provide backwards compatibility via a plugin that sets the commands/BBCodes to the default ones if none specified.
Consider making the editor a Web Component #
This would make the editor more self-contained and better encapsulate HTML/CSS via the shadow DOM API. All browsers that support ES modules also support Web Components, so support shouldn't be an issue.
Using Web Components could also be used to allow creating the editor declaritavley:
Although there is no nice way to pass JS to web components declaratively, only pragmatically, so would likely conflict with .
Changes to core #
Move emoticons to a plugin #
Not all users need emoticon support, so it would be better to move them into a plugin instead.
Can have backwards compatibility plugin autoload the plugin.
Move undo/redo into the core #
As undo/redo is a common operation so probably worth moving. It would give a much better out-of-box UX.
Can have backwards compatibility plugin which would simply ignore the undo plugin if specified.
Improve shortcuts / allow remapping #
Would need to give commands a title/descriptions and allow mapping shortcuts to command IDs.
Could then make a new command/plugin that can be used to display shortcuts and re-map commands to new shortcuts.
This would give a better UX on non-Latin keyboard layouts as currently triggering a default shortcut requires typing Latin characters.
This would also allow multiple aliases support which could help with typing certain shortcuts (like
ctrl+1
that also needs to havectrl+shift+1
for some French keyboard layouts).Change code behaviour #
Currently the
<code>
tag has a lot of special exceptions. They should be made configurable to all tags. It would also be better to change to using<pre><code>
and store it as<pre data-sce-code="true">
while editing in WYSIWYG.Not really possible to provide any backwards compatibility for this.
API changes #
Rename bind/unbind to on/off #
Rename bind/unbind to on/off as matches what most other JS libraries use.
Can offer backwards compatibility easily by just aliasing them in a backwards compatibility plugin.
Remove event handler methods like keypress() #
Remove the helper event methods like keypress, not much more ergonomic than on/off and increase the API's size.
Can offer backwards compatibility easily via backwards compatibility plugin.
Rename rangeHelper insertHTML() #
Rename the rangeHelper
insertHTML()
method toinsertHtml()
to match naming of other methods.Can provide backwards compatibility by aliasing it in a backwards compatibility plugin.
Reduce exposed methods #
The utils
each
method can be replaced withforEach
andArray.from
, which are now available in all supported browsers.It might also be worth reducing or outright removing the exposed dom.* functions.
Most of them are either very editor specific or can be done using the native APIs fairly easily.
This would make internal refactoring easier.
Remove
bbcode.isPreFormatted
#Alias of skipLastLineBreak which only exists for backwards compatibility. Isn't documented so unlikely anybody is still using it and easy to migrate if anyone is.
Can offer backwards compatibility easily via backwards compatibility plugin.
Add Typescript definitions #
Add Typescript definitions to the package.
Improve table UI #
Improve table editing UI. It should have a way to add or remove rows and columns easily.
Would also be good to update the insert menu to use the drag and drop UI which is common:
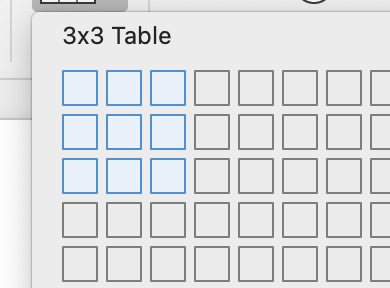
Use real buttons in toolbar #
Use real
<button>
elements in toolbar. Better for accessibility and the right element to use. No way to make this backwards compatible.Add support for self-closing slash in BBCodes #
Can sort of be done currently if there are no attributes but if there are any attributes, it will need to be proper support to work. This can default to current behaviour so can be backwards compatible.
Switch to Prettier formatting #
Switch to using Prettier to auto-format the code. Fully backwards compatible and makes it easier to contribute code as contributors don't need to think about formatting.
Beta Was this translation helpful? Give feedback.
All reactions