You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Copy file name to clipboardexpand all lines: README.md
+2
Original file line number
Diff line number
Diff line change
@@ -7,6 +7,8 @@ The library provides fully typed support for all homie5 datatypes.
7
7
8
8
Due to this, the usage of the library is a bit more involved as with a completly ready to use homie library. Benefit is however that you can use the library basically everywhere from a simple esp32, raspberrypi to a x86 machine.
9
9
10
+
[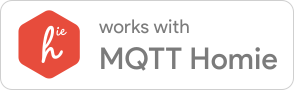](https://homieiot.github.io/)
11
+
10
12
## Content
11
13
12
14
<!-- TOC start (generated with https://github.com/derlin/bitdowntoc) -->
/// Provides generators for all mqtt subscribe and publish packages needed for a homie5 controller
11
-
/// ### The general order for discovering devices is as follows:
12
-
/// * Start with the Subscriptions returned by [`Homie5ControllerProtocol::discover_devices`]
13
-
/// This will subscribe to the $state attribute of all devices.
14
-
/// * When receiving a [`Homie5Message::DeviceState`] message, check if the device is already
15
-
/// known, if not subscribe to the device using [`Homie5ControllerProtocol::subscribe_device`].
16
-
/// This will subscibe to all the other device attributes like $log/$description/$alert
17
-
/// * When receiving a [`Homie5Message::DeviceDescription`] message, store the description for the
18
-
/// device and subscibe to all the property values using
19
-
/// [`Homie5ControllerProtocol::subscribe_props`]
20
-
/// * after this you will start receiving [`Homie5Message::PropertyValue`] and
21
-
/// ['Homie5Message::PropertyTarget`] messages for the properties of the device
28
+
/// The `Homie5ControllerProtocol` struct provides the core functionality for generating MQTT subscription and publish commands required for interacting with Homie 5 devices.
29
+
///
30
+
/// This struct simplifies the process of discovering devices, subscribing to device attributes, handling device property changes, and sending commands or broadcasts to devices in the Homie MQTT protocol. It supports managing device discovery, subscriptions to device states and properties, and sending set commands and broadcast messages.
31
+
///
32
+
/// The `Homie5ControllerProtocol` is designed for use in Homie-based IoT controllers where efficient communication with multiple devices is required. It offers a straightforward interface for subscribing to device attributes and managing device properties.
33
+
///
34
+
/// # Usage
35
+
///
36
+
/// To create an instance of `Homie5ControllerProtocol`, use the `new` method. This struct provides methods for discovering devices, subscribing to device attributes and properties, and sending commands or broadcasts via MQTT topics.
37
+
///
38
+
/// # Example
39
+
///
40
+
/// ```rust
41
+
/// use homie5::{Homie5ControllerProtocol, HomieDomain};
42
+
///
43
+
/// let protocol = Homie5ControllerProtocol::new();
44
+
/// let subscriptions = protocol.discover_devices(&HomieDomain::Default);
45
+
/// for subscription in subscriptions {
46
+
/// // Handle each subscription
47
+
/// }
48
+
/// ```
49
+
///
50
+
/// The struct is intended for use in controller applications interacting with multiple Homie devices, enabling efficient subscription management and MQTT communication.
22
51
#[derive(Default)]
23
52
pubstructHomie5ControllerProtocol{}
24
53
25
54
implHomie5ControllerProtocol{
26
-
/// Create a new Homie5ControllerProtocol object
55
+
/// Creates a new `Homie5ControllerProtocol` instance.
56
+
///
57
+
/// # Returns
58
+
/// A new `Homie5ControllerProtocol` object initialized with default values.
27
59
pubfnnew() -> Self{
28
60
Default::default()
29
61
}
30
62
63
+
/// Generates a subscription to discover Homie devices by subscribing to the `$state` attribute of all devices.
64
+
///
65
+
/// # Parameters
66
+
/// - `homie_domain`: The Homie domain in which to discover devices.
67
+
///
68
+
/// # Returns
69
+
/// An iterator over a `Subscription` object that subscribes to the `$state` attribute of all devices in the specified domain.
0 commit comments