|
| 1 | +# Promises and fetch |
| 2 | + |
| 3 | +--- |
| 4 | + |
| 5 | +## What is a promise? |
| 6 | + |
| 7 | +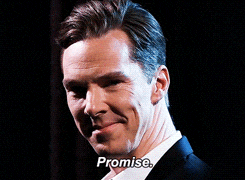 |
| 8 | + |
| 9 | +--- |
| 10 | + |
| 11 | +Coined by Barbara Liskov |
| 12 | + |
| 13 | + |
| 14 | + |
| 15 | +--- |
| 16 | + |
| 17 | +and Liuba Shrira in 1988. |
| 18 | + |
| 19 | + |
| 20 | + |
| 21 | +--- |
| 22 | + |
| 23 | +A promise is a **special JavaScript object** that may produce a single value some time in the future: |
| 24 | +- either a resolved value, |
| 25 | +- or a reason that it’s not resolved (e.g., a network error occurred). |
| 26 | + |
| 27 | + |
| 28 | +--- |
| 29 | + |
| 30 | +A promise links a “producing code” and a “consuming code” together. |
| 31 | +- **producing code**: code that takes time and produces a promised result. |
| 32 | +- **consuming code**: waits for result of the producing code and does something with it. |
| 33 | + |
| 34 | + |
| 35 | +--- |
| 36 | + |
| 37 | +## constructor syntax :construction_worker: |
| 38 | +```JavaScript |
| 39 | +let promise = new Promise(function(resolve, reject) { |
| 40 | + // executor (the producing code, "singer") |
| 41 | +}); |
| 42 | +``` |
| 43 | + |
| 44 | +--- |
| 45 | + |
| 46 | + |
| 47 | +The promise object returned by new Promise constructor has internal properties |
| 48 | +- **state** — initially "pending", then changes to either "fulfilled" when resolve is called or "rejected" when reject is called. |
| 49 | +- **result** — initially undefined, then changes to value when resolve(value) called or error when reject(error) is called. |
| 50 | + |
| 51 | + |
| 52 | +--- |
| 53 | + |
| 54 | + |
| 55 | + |
| 56 | + |
| 57 | + |
| 58 | +--- |
| 59 | + |
| 60 | +A pending promise may transition into a fulfilled or rejected state. |
| 61 | + |
| 62 | +Once settled, it must have a value that must not change and cannot be resettled. |
| 63 | + |
| 64 | +Calling resolve() or reject() again will have no effect. |
| 65 | + |
| 66 | +The immutability of a settled promise is an important feature. |
| 67 | + |
| 68 | +--- |
| 69 | + |
| 70 | +Promises are eager... |
| 71 | + |
| 72 | +--- |
| 73 | + |
| 74 | +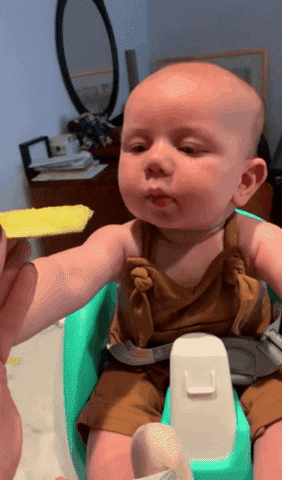 |
| 75 | + |
| 76 | +--- |
| 77 | + |
| 78 | +Will start doing whatever task you give it as soon as the promise constructor is invoked. |
| 79 | + |
| 80 | + |
| 81 | +That means the executor runs automatically once the promise is created. The executor should do a job, then call resolve or reject to change the state of the corresponding promise object. |
| 82 | + |
| 83 | + |
| 84 | +--- |
| 85 | + |
| 86 | +## Promises vs callbacks: Advantages :+1: |
| 87 | + |
| 88 | + |
| 89 | +--- |
| 90 | + |
| 91 | + |
| 92 | +**Readability** |
| 93 | +- they reduce the amount of nested code |
| 94 | +- they allow you to visualize the execution flow easily |
| 95 | + |
| 96 | +--- |
| 97 | + |
| 98 | + |
| 99 | + |
| 100 | +**Error handling**: let you handle all errors at once at the end of the chain |
| 101 | + |
| 102 | + |
| 103 | + |
| 104 | +--- |
| 105 | + |
| 106 | + |
| 107 | +**Simultaneous callbacks**: with Promise.all you can fire off two (or many) promises at the same time if the operations aren’t dependent on each other, but both results are needed to perform a third action. |
| 108 | + |
| 109 | + |
| 110 | + |
| 111 | +--- |
| 112 | + |
| 113 | +```Javascript |
| 114 | +const friesPromise = getFries() |
| 115 | +const burgerPromise = getBurger() |
| 116 | +const drinksPromise = getDrinks() |
| 117 | + |
| 118 | +const eatMeal = Promise.all([ |
| 119 | + friesPromise, |
| 120 | + burgerPromise, |
| 121 | + drinksPromise |
| 122 | +]) |
| 123 | + .then([fries, burger, drinks] => { |
| 124 | + console.log(`Chomp. Awesome ${burger}! 🍔`) |
| 125 | + console.log(`Chomp. Delicious ${fries}! 🍟`) |
| 126 | + console.log(`Slurp. Ugh, shitty drink ${drink} 🤢 `) |
| 127 | + }) |
| 128 | +``` |
| 129 | + |
| 130 | + |
| 131 | +--- |
| 132 | + |
| 133 | + |
| 134 | +## Promises vs callbacks: Disadvantages :-1: |
| 135 | + |
| 136 | + |
| 137 | +--- |
| 138 | + |
| 139 | + |
| 140 | +There's nothing promises can do that callbacks can't do, but there are things that promises can't do, such as: |
| 141 | + |
| 142 | +- Synchronous notifications. |
| 143 | +- Notifications that may occur more than once (need to call the callback more than once). Promises are one-shot devices and cannot be used on repeat. |
| 144 | + |
| 145 | + |
| 146 | +--- |
| 147 | + |
| 148 | + |
| 149 | +Running code that contains promises can be slower than running code that is using callbacks, this might improve over time though. |
| 150 | + |
| 151 | + |
| 152 | +--- |
| 153 | + |
| 154 | +## Promises vs (async/await): Disadvantages :-1: |
| 155 | + |
| 156 | + |
| 157 | +--- |
| 158 | + |
| 159 | + |
| 160 | +There is a new kid in town: async/await. It's syntactic sugar on top of promises but in some cases it works really well. (There's a lot of debate!) |
| 161 | + |
| 162 | +--- |
| 163 | + |
| 164 | +Example using promises: |
| 165 | + |
| 166 | +```javascript= |
| 167 | +const makeRequest = () => |
| 168 | + getJSON() |
| 169 | + .then(data => { |
| 170 | + console.log(data) |
| 171 | + return "done" |
| 172 | + }) |
| 173 | +
|
| 174 | +makeRequest() |
| 175 | +``` |
| 176 | + |
| 177 | +--- |
| 178 | + |
| 179 | +Same example using async/await: |
| 180 | +```javascript= |
| 181 | +const makeRequest = async () => { |
| 182 | + console.log(await getJSON()) |
| 183 | + return "done" |
| 184 | +} |
| 185 | +
|
| 186 | +makeRequest() |
| 187 | +``` |
| 188 | + |
| 189 | + |
| 190 | +--- |
| 191 | + |
| 192 | + |
| 193 | + |
| 194 | +## What is fetch? |
| 195 | + |
| 196 | +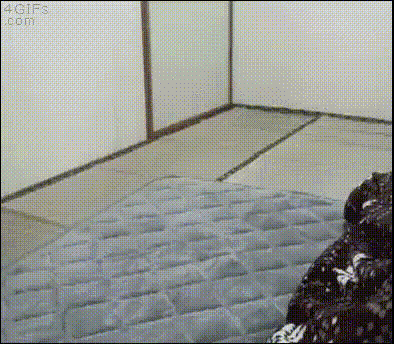 |
| 197 | + |
| 198 | +--- |
| 199 | + |
| 200 | +The Fetch API is a web API that provides an interface for fetching resources asynchronously (API requests). It will seem familiar to anyone who has used XMLHttpRequest, but the new API provides a more powerful and flexible feature set. |
| 201 | + |
| 202 | +--- |
| 203 | + |
| 204 | + |
| 205 | + |
| 206 | +It has good support across the major browsers, except IE. |
| 207 | + |
| 208 | +It's for front end API calls but you can use node-fetch on the backend. |
| 209 | + |
| 210 | + |
| 211 | +--- |
| 212 | + |
| 213 | +## How do you use it? |
| 214 | + |
| 215 | +* The fetch() method takes one mandatory argument - the path to the resource you want to fetch |
| 216 | +* It returns a Promise that resolves to the Response to that request, whether it is successful or not |
| 217 | +* You can also optionally pass in an init options object as the second argument (see Request). |
| 218 | + |
| 219 | +--- |
| 220 | + |
| 221 | +#### Example |
| 222 | + |
| 223 | +```javascript= |
| 224 | +fetch('http://example.com/movies.json') |
| 225 | + .then(function(response) { |
| 226 | + return response.json(); |
| 227 | + }) |
| 228 | + .then(function(myJson) { |
| 229 | + console.log(JSON.stringify(myJson)); |
| 230 | + }); |
| 231 | +``` |
| 232 | + |
| 233 | +--- |
| 234 | + |
| 235 | +## Fetch: advantages and disadvantages |
| 236 | + |
| 237 | +--- |
| 238 | + |
| 239 | +#### Advantages: |
| 240 | + |
| 241 | +* Simpler syntax |
| 242 | +* Promise based - meaning it's easier to write cleaner asynchronous code. |
| 243 | +* It has a neat definition for what a request is, and what a response is. |
| 244 | + |
| 245 | +--- |
| 246 | + |
| 247 | +  |
| 248 | + |
| 249 | +--- |
| 250 | + |
| 251 | + |
| 252 | +#### Disadvantages: |
| 253 | + |
| 254 | +* By default, it doesn’t send cookies might be an initial source of confusion to some. Authentication needs to be handled manually. |
| 255 | + |
| 256 | + |
| 257 | + |
| 258 | + |
| 259 | + |
| 260 | +--- |
| 261 | + |
| 262 | +## Resources |
| 263 | + |
| 264 | +* https://medium.com/javascript-scene/master-the-javascript-interview-what-is-a-promise-27fc71e77261 |
| 265 | +* https://www.quora.com/What-are-the-benefits-of-the-Fetch-API-over-AJAX-xmlhttprequest |
| 266 | +* https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch |
| 267 | + |
| 268 | +--- |
| 269 | + |
| 270 | +* https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API |
| 271 | +* https://flaviocopes.com/fetch-api/ |
| 272 | +* https://hackernoon.com/6-reasons-why-javascripts-async-await-blows-promises-away-tutorial-c7ec10518dd9 |
| 273 | +* https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch |
| 274 | + |
| 275 | +--- |
0 commit comments