|
| 1 | +# Session-management in Express |
| 2 | + |
| 3 | +--- |
| 4 | + |
| 5 | +## What are sessions? |
| 6 | + |
| 7 | +- By default an **HTTP request cannot remember** or keep track **of** what **requests** came **before** it. |
| 8 | + |
| 9 | + |
| 10 | +- But sometimes its necessary for both the client and server to **remember the state** when sending requests and returning responses(**eg: when a user is logged in**). |
| 11 | + |
| 12 | +--- |
| 13 | + |
| 14 | +#### Stateful and stateless |
| 15 | + |
| 16 | +- Last week we talked about **stateful vs stateless** authentication, and we implemented stateless authentication with JWTs. |
| 17 | + |
| 18 | +- the user's state(in our projects this was logged_in=true) is stored in the JWT on the **CLIENT SIDE** |
| 19 | + |
| 20 | +#### What does the browser do?(stateless) |
| 21 | + |
| 22 | +- It **VERIFIES** that these details have not been tampered with **(the state is never stored on the server)** |
| 23 | + |
| 24 | +--- |
| 25 | + |
| 26 | +#### Is there another way to do this? |
| 27 | + |
| 28 | +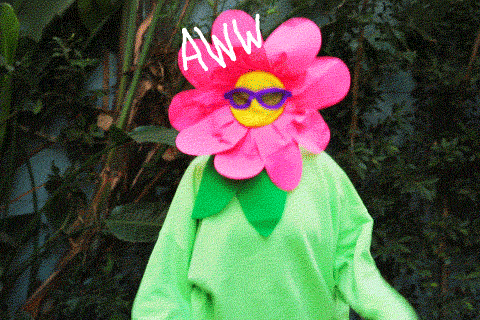 |
| 29 | + |
| 30 | + |
| 31 | +--- |
| 32 | + |
| 33 | +### Stateful Authentication |
| 34 | +- The other way to do this is by **storing the client's state**(logged_in boolean or anything else really) **on the SERVER** |
| 35 | + |
| 36 | +--- |
| 37 | + |
| 38 | +#### Well, Simple explanation(stateful) |
| 39 | +1) A **session id**(which is usually a string or a number) is **sent as a cookie** to the client |
| 40 | +2) The session id is a **reference to** the state data, remember that this data is **stored on the server** |
| 41 | +3) Every time the client makes a request, the session id **is sent as a header**, and the **server looks for** the user state **matching** that session id. |
| 42 | + |
| 43 | +--- |
| 44 | + |
| 45 | +### This is what sessions are! |
| 46 | +- A way to identify the source of a request |
| 47 | +- And provide the corresponding responses. |
| 48 | + |
| 49 | +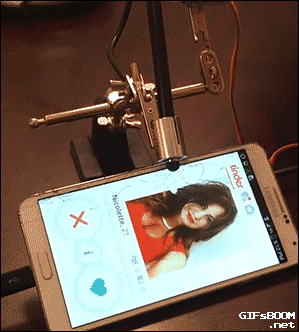 |
| 50 | + |
| 51 | + |
| 52 | +--- |
| 53 | + |
| 54 | + |
| 55 | +## What are the different ways of managing sessions in express? |
| 56 | + |
| 57 | + |
| 58 | +--- |
| 59 | + |
| 60 | +## Cookie-session |
| 61 | + |
| 62 | + |
| 63 | +Cookie-session is a simple / lightweight cookie-based session implementation. |
| 64 | + |
| 65 | + |
| 66 | +--- |
| 67 | + |
| 68 | +This module stores the session data on the client within a cookie, while a module like express-session stores only a session identifier on the client within a cookie and stores the session data on the server, typically in a database. |
| 69 | + |
| 70 | + |
| 71 | +--- |
| 72 | + |
| 73 | + |
| 74 | +### Cookie-session |
| 75 | + |
| 76 | +* does not require any database / resources on the server side, though the total session data cannot exceed the browser's max cookie size, |
| 77 | +* can simplify certain load-balanced scenarios, |
| 78 | + |
| 79 | +--- |
| 80 | + |
| 81 | + #### How do I install it |
| 82 | +` |
| 83 | +$ npm install cookie-session |
| 84 | +` |
| 85 | + |
| 86 | + |
| 87 | + |
| 88 | +--- |
| 89 | + |
| 90 | + |
| 91 | +```javascript |
| 92 | +var cookieSession = require('cookie-session') |
| 93 | +var express = require('express') |
| 94 | + |
| 95 | +var app = express() |
| 96 | + |
| 97 | +app.use(cookieSession({ |
| 98 | + name: 'session', |
| 99 | + keys: [insert secret keys] |
| 100 | +})) |
| 101 | + |
| 102 | +app.use(function (req, res, next) { |
| 103 | + var n = req.session.views || 0 |
| 104 | + req.session.views = n++ |
| 105 | + res.end(n + ' views') |
| 106 | +}) |
| 107 | + |
| 108 | +app.listen(3000) |
| 109 | +``` |
| 110 | +keys refer to secrets to sign your cookie with. |
| 111 | +cookieSession automatically generates your sessiondata, signs it with the secret, and stores it in a global session object |
| 112 | + |
| 113 | + |
| 114 | + |
| 115 | + |
| 116 | + |
| 117 | +--- |
| 118 | + |
| 119 | +### Using the `express-session` module |
| 120 | + |
| 121 | +Stateful session management, so only user identifier (session id) is stored in a cookie and actual session data is stored in one of the storage options. |
| 122 | + |
| 123 | +requires a secret, and the sessionid is signed(encrypted) before setting the cookie, and decrypted when the server is checking for a session. |
| 124 | + |
| 125 | +--- |
| 126 | + |
| 127 | +```javascript |
| 128 | +cont session=require('express-session') |
| 129 | +app.use(session({secret:'fac17'})); |
| 130 | + |
| 131 | +``` |
| 132 | + |
| 133 | +--- |
| 134 | + |
| 135 | +`express-session` middleware inserts another object into `req` object called `session`, that will be available for each request |
| 136 | +We can manipulate like any other object |
| 137 | + |
| 138 | +``` |
| 139 | +req.session.whatever='you want'; |
| 140 | +``` |
| 141 | + |
| 142 | +--- |
| 143 | + |
| 144 | +#### Different Stores |
| 145 | + |
| 146 | +by default MemoryStore but there are others include: session-file-store, express-mysql-session, connect-redis |
| 147 | + |
| 148 | +```javascript |
| 149 | +const session=require('express-session'); |
| 150 | +const fileStore=require('session-file-store')(session) |
| 151 | +app.use(session( { secret:'fac17', store:fileStore( {path:'./sessions'}) })) |
| 152 | +``` |
| 153 | + |
| 154 | +--- |
| 155 | + |
| 156 | +## Create a minimal example of how to set up a session: the counter |
| 157 | + |
| 158 | +--- |
| 159 | + |
| 160 | +#### When a user visits the site: |
| 161 | +- It creates a new session for the user |
| 162 | +- Assigns them a cookie |
| 163 | +- Next time the user comes |
| 164 | +- The cookie is checked |
| 165 | +- The page_view session variable is updated accordingly |
| 166 | + |
| 167 | + |
| 168 | +--- |
| 169 | + |
| 170 | +```javascript |
| 171 | +const express = require('express'); |
| 172 | +const session = require('express-session'); |
| 173 | +const app = express(); |
| 174 | +``` |
| 175 | + |
| 176 | +--- |
| 177 | + |
| 178 | +```javascript= |
| 179 | +// initiate session middleware |
| 180 | +app.use(session({secret: "Shh, its a secret!"})); |
| 181 | +
|
| 182 | +app.get('/', function(req, res){ |
| 183 | +
|
| 184 | + // req.session is preserved between requests |
| 185 | + if(req.session.page_views){ |
| 186 | + req.session.page_views++; |
| 187 | + res.send("You visited this page " + req.session.page_views + " times"); |
| 188 | + } else { |
| 189 | + req.session.page_views = 1; |
| 190 | + res.send("Welcome to this page for the first time!"); |
| 191 | + } |
| 192 | +}); |
| 193 | +app.listen(3000); |
| 194 | +
|
| 195 | +``` |
| 196 | + |
| 197 | +--- |
| 198 | + |
| 199 | +Now if you run the app and go to localhost:3000, the following output will be displayed. |
| 200 | + |
| 201 | + |
| 202 | + |
| 203 | +--- |
| 204 | + |
| 205 | +If you revisit the page, the page counter will increase. The page in the following screenshot was refreshed 42 times. |
| 206 | + |
| 207 | + |
| 208 | + |
| 209 | +--- |
| 210 | + |
| 211 | +## RESOURCES |
| 212 | +[manage-session-using-node-js-express](https://codeforgeek.com/manage-session-using-node-js-express-4/) |
| 213 | +[express session](https://www.tutorialspoint.com/expressjs/expressjs_sessions.htm) |
| 214 | +[The polyglote deveoper](https://www.thepolyglotdeveloper.com/2015/01/session-management-expressjs-web-application/) |
| 215 | + |
| 216 | +https://flaviocopes.com/express-sessions/ |
| 217 | + |
| 218 | +https://dzone.com/articles/securing-nodejs-managing-sessions-in-expressjs |
| 219 | + |
| 220 | +https://www.npmjs.com/package/cookie-session |
0 commit comments