-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
54f4496
commit 3741314
Showing
6 changed files
with
316 additions
and
97 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1 +1,2 @@ | ||
.idea/ | ||
.idea/ | ||
/vendor/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,7 +1,7 @@ | ||
# [PHP] Ulam Spiral Generator | ||
### PHP 5.6+ / PHP 7 | ||
### PHP 7.2.5+ | ||
|
||
Current version: 1.0 | ||
Current version: 1.1 (2022-04-23) | ||
|
||
Mathematic Ulam spiral generator and renderer with programmable callbacks written in PHP. | ||
|
||
|
@@ -14,48 +14,48 @@ from https://en.wikipedia.org/wiki/Ulam_spiral: | |
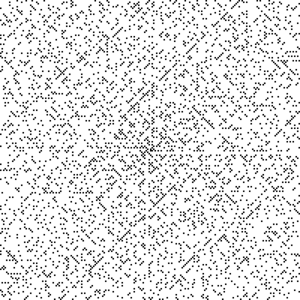 | ||
|
||
|
||
**Install with composer:** | ||
``` | ||
composer require szczyglis/php-ulam-spiral-generator | ||
``` | ||
|
||
## Features: | ||
|
||
- Ulam spiral matrix builder working with any dataset | ||
- Built in on-screen spiral renderer (as HTML table or raw data) | ||
- For standalone and external usage (it is only one PHP class) | ||
- Easy to use | ||
- Programmable callbacks for numbers highlighting and counting in rows and columns | ||
- Javascript-based real time rows/columns/crosses highlighter | ||
- Programmable callbacks for in rows and columns number highlighting and counting | ||
- Javascript-based real-time rows/columns/crosses highlighter | ||
|
||
|
||
## Usage example: | ||
``` | ||
```php | ||
<?php | ||
|
||
include __DIR__.'/UlamSpiral.php'; | ||
/* Create config (optional) */ | ||
$config = [ | ||
'raw' => false, | ||
'append_css' => true, | ||
'append_js' => true, | ||
'no_append_jquery' => false, | ||
'counters_mode' => 'sum', | ||
'row_counters' => true, | ||
'col_counters' => true, | ||
'cell_width' => 35, | ||
'cell_height' => 35, | ||
'cell_font_size' => 12 | ||
require __DIR__ . '/vendor/autoload.php'; | ||
|
||
use Szczyglis\UlamSpiralGenerator\UlamSpiral; | ||
|
||
// configuration | ||
$config = [ | ||
'raw' => false, // if true then displays raw spiral (without CSS) | ||
'append_css' => true, // enables CSS stylizing | ||
'append_js' => true, // enables JS features | ||
'no_append_jquery' => false, // disables jQuery script appending if true | ||
'counters_mode' => 'count', // sets counters mode (sum of values or occurencies count) | ||
'row_counters' => true, // enables vertical counters | ||
'col_counters' => true, // enables horizontal counters | ||
'cell_width' => 35, // sets width of cell in pixels, | ||
'cell_height' => 35, // sets height of cell in pixels | ||
'cell_font_size' => 12, // sets font size in pixels | ||
]; | ||
|
||
$dataset = range(1, 1000); // create dataset | ||
|
||
/* Create new generator */ | ||
$ulam = new UlamSpiral($config); | ||
/* Add dataset */ | ||
$ulam->dataset = range(1, 1000); | ||
/* Add custom callbacks for counters and markers (optional) */ | ||
$ulam->addCounter('sum', function($value) { | ||
$ulam = new UlamSpiral($config); // create new generator | ||
$ulam->setDataset($dataset); // define dataset | ||
$ulam->addCounter('sum', function($value) { // add custom callbacks for counters ( optional ) | ||
return true; | ||
}); | ||
$ulam->addCounter('prime', function($value) { | ||
|
@@ -65,25 +65,23 @@ $ulam->addCounter('prime', function($value) { | |
} | ||
} | ||
}); | ||
$ulam->addMarker('prime', function($value) { | ||
$ulam->addMarker('prime', function($value) { // add custom callbacks for markers ( optional ) | ||
if (is_integer($value)) { | ||
if (UlamSpiral::isPrime($value)) { | ||
return '#e9e9e9'; | ||
} | ||
} | ||
}); | ||
|
||
/* Build Ulam spiral matrix */ | ||
$ulam->buildMatrix(); | ||
/* $ulam->matrix is created now. */ | ||
$ulam->buildMatrix(); // build Ulam spiral matrix | ||
echo $ulam->render(); // render spiral | ||
|
||
/* Render spiral */ | ||
echo $ulam->render(); | ||
$matrix = $ulam->getMatrix(); // returns spiral's matrix | ||
|
||
``` | ||
You can use any PHP array filled with numbers or chars in `$ulam->dataset`, all values from array will be placed on spiral. | ||
You can use any PHP array filled by numbers/or chars in `$ulam->dataset`, all values from array will be placed on spiral. | ||
|
||
After execute`$ulam->buildMatrix()`, the matrix created by this method is always available in array `$ulam->matrix`, x and y coords of placed on spiral values are available in array `$ulam->coords`. | ||
After execute`$ulam->buildMatrix()`, the matrix created by this method will be available in `$ulam->matrix` array, x and y coords accorded to placed on spiral values will be available in `$ulam->coords` array. You have access to matrix with `$ulam->getMatrix()` method. | ||
|
||
## Screenshots: | ||
|
||
|
@@ -100,42 +98,46 @@ Raw version: | |
|
||
## Repository includes: | ||
|
||
- UlamSpiral.php - base class | ||
- example.php - usage example | ||
- `src/PhpUlamSpiral.php` - base class | ||
|
||
- `example.php` - usage example | ||
|
||
## Configuration: | ||
|
||
You can configure generator by creating `$config` array and put it into constructor. | ||
All keys in array are described below: | ||
|
||
`raw` (bool) - `[true|false]` display raw spiral (without CSS), default: `false` | ||
All keys in an array are described below: | ||
|
||
`raw` (bool) - `[true|false]` if true then displays raw spiral (without CSS), default: `false` | ||
|
||
`append_css` (bool) - `[true|false]` enable CSS, default: `true` | ||
`append_css` (bool) - `[true|false]` enables CSS, default: `true` | ||
|
||
`append_js` (bool) - `[true|false]` enable JS, default: `true` | ||
`append_js` (bool) - `[true|false]` enables JS, default: `true` | ||
|
||
`no_append_jquery` (bool) `[true|false]` - disable jQuery script appending, default: `false` | ||
`no_append_jquery` (bool) `[true|false]` - disables jQuery script appending, default: `false` | ||
|
||
`counters_mode` (string) `[sum|count]` - counters mode (sum of values or occurencies count), default: `count` | ||
`counters_mode` (string) `[sum|count]` - sets counters mode (sum of values or occurencies count), default: `count` | ||
|
||
`row_counters` (bool) `[true|false]` - enable vertical counters, default: `true` | ||
`row_counters` (bool) `[true|false]` - enables vertical counters, default: `true` | ||
|
||
`col_counters` (bool) `[true|false]` - enable horizontal counters, default: `true` | ||
`col_counters` (bool) `[true|false]` - enables horizontal counters, default: `true` | ||
|
||
`cell_width` (int) - cell width in pixels, default: `35` | ||
`cell_width` (int) - sets width of cell in pixels, default: `35` | ||
|
||
`cell_height` (int) - cell height in pixels, default: `35` | ||
`cell_height` (int) - sets height of cell in pixels, default: `35` | ||
|
||
`cell_font_size` (int) - font size in pixels, default: `15` | ||
`cell_font_size` (int) - sets font size in pixels, default: `15` | ||
|
||
|
||
## Custom callbacks: | ||
## Defining custom callbacks: | ||
|
||
### Numbers highlighting | ||
|
||
You can create your own marker callback for highlight specified numbers (e.g. prime numbers, even numbers, numbers greater than specified one, etc.). Callback takes one argument with current number and must return `HTML color code` with which to highlight. If callback returns `null` or `false` then number will not be affected. You can create as many markers as you like, each one for a different number type. | ||
|
||
Example showing how to create marker callback for even numbers: | ||
``` | ||
Example shows how to create marker callback for even numbers: | ||
|
||
```php | ||
$ulam = new UlamSpiral(); | ||
$ulam->addMarker('even', function($value) { | ||
if (is_integer($value)) { | ||
|
@@ -159,8 +161,9 @@ $ulam->addMarker('even', function($value) { | |
|
||
Counter callbacks are for creating counters in spiral headers (horizontal and vertical). Counters can count specific numbers in row or column and display a result in row or column header. There are 2 types of counters: `count` and `sum`. You can choose behaviour in config. First type - `count` counts all occurencies of specified type of numbers in row or column, second type: `sum` displays sum of their values. If callback returns `true` then number will be affected by counter. you can create as many counters as you like, each one for a different number type. | ||
|
||
Example showing how to create counter callback for even numbers: | ||
``` | ||
Example shows how to create counter callback for even numbers: | ||
|
||
```php | ||
$ulam = new UlamSpiral(); | ||
$ulam->addCounter('even', function($value) { | ||
if (is_integer($value)) { | ||
|
@@ -186,6 +189,11 @@ $ulam->addCounter('even', function($value) { | |
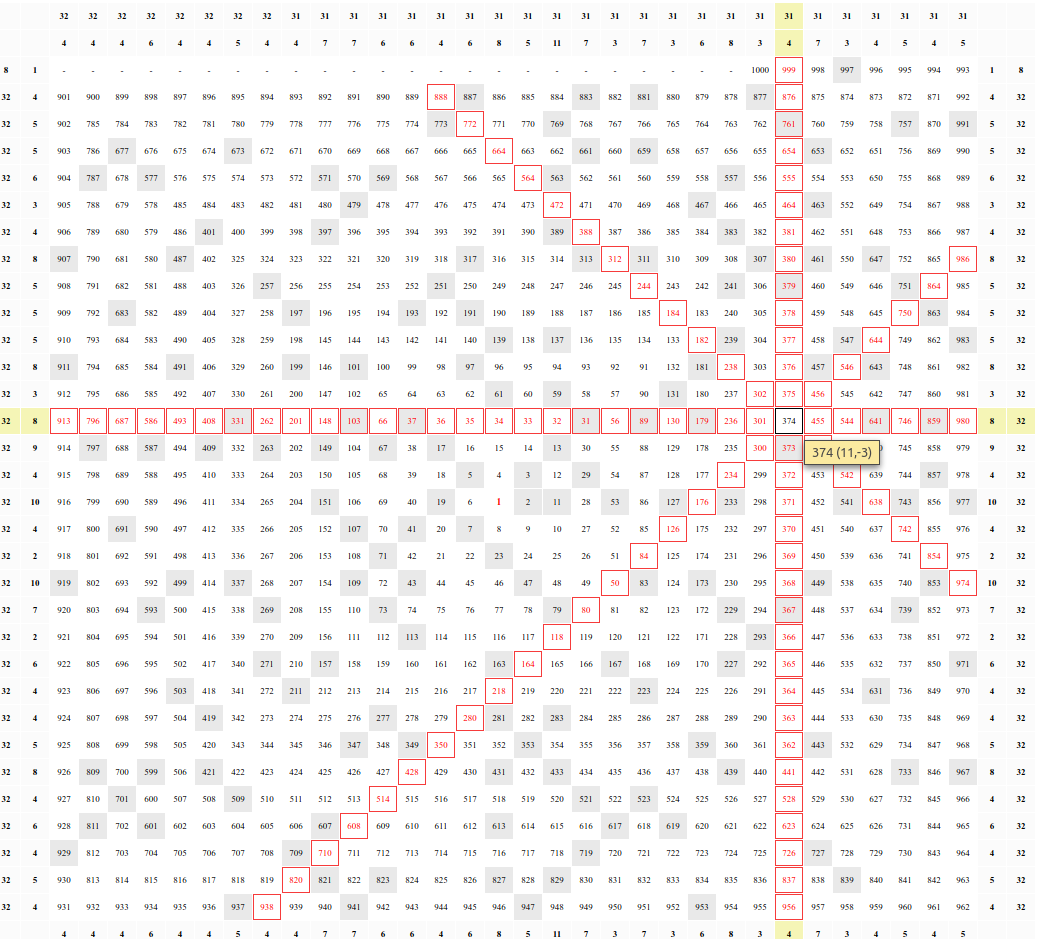 | ||
|
||
|
||
### Changelog | ||
|
||
- `v1.1` -- package was added to packagist (2022-04-23) | ||
|
||
|
||
### Ulam Spiral Generator is free to use but if you liked then you can donate project via BTC: | ||
|
||
**14X6zSCbkU5wojcXZMgT9a4EnJNcieTrcr** | ||
|
@@ -194,11 +202,14 @@ or by PayPal: | |
**[https://www.paypal.me/szczyglinski](https://www.paypal.me/szczyglinski)** | ||
|
||
|
||
Enjoy! | ||
|
||
**Enjoy!** | ||
|
||
MIT License | 2020 Marcin 'szczyglis' Szczygliński | ||
MIT License | 2022 Marcin 'szczyglis' Szczygliński | ||
|
||
https://github.com/szczyglis-dev/php-ulam-spiral-generator | ||
|
||
https://szczyglis.dev/ulam-spiral-generator | ||
|
||
https://szczyglis.dev | ||
|
||
Contact: [email protected] |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
{ | ||
"name": "szczyglis/php-ulam-spiral-generator", | ||
"description": "Mathematic Ulam spiral generator and renderer with programmable callbacks written in PHP.", | ||
"type": "library", | ||
"license": "MIT", | ||
"homepage": "https://github.com/szczyglis-dev/php-ulam-spiral-generator", | ||
"autoload": { | ||
"psr-4": { | ||
"Szczyglis\\UlamSpiralGenerator\\": "src/" | ||
} | ||
}, | ||
"keywords": [ | ||
"math", | ||
"mathematic", | ||
"ulam-spiral", | ||
"ulam", | ||
"generator", | ||
"alghoritm", | ||
"prime numbers" | ||
], | ||
"authors": [ | ||
{ | ||
"name": "szczyglis", | ||
"email": "[email protected]", | ||
"homepage": "https://szczyglis.dev" | ||
} | ||
], | ||
"minimum-stability": "stable", | ||
"require": { | ||
"php": ">=7.2.5" | ||
} | ||
} |
Some generated files are not rendered by default. Learn more about how customized files appear on GitHub.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.